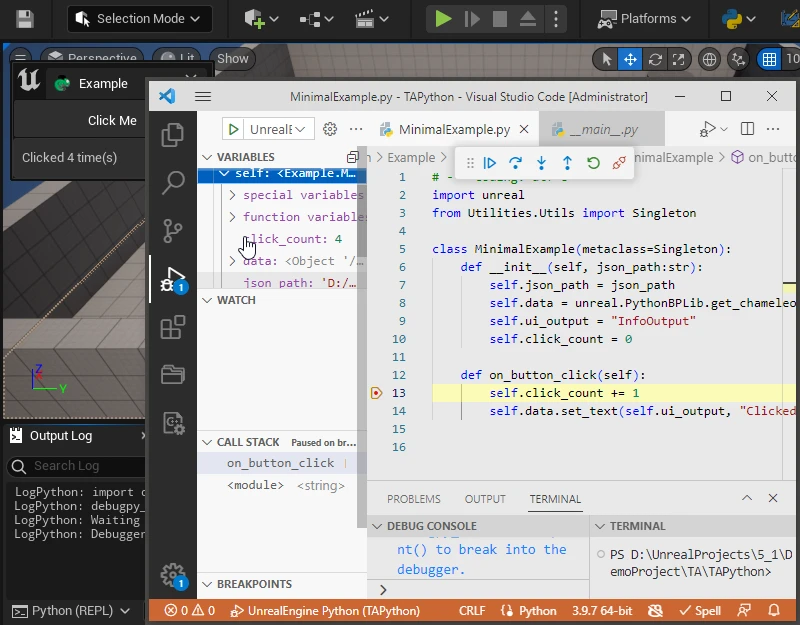
When debugging Python code, you can follow these steps to perform breakpoint debugging in VSCode. The official Python plugin also provides step-by-step instructions in <UE_Root>\Engine\Plugins\Experimental\PythonScriptPlugin\Content\Python\debugpy_unreal.py
:
- import debugpy_unreal into Python within the UE Editor.
- If debugpy has not yet been installed, run debugpy_unreal.install_debugpy().
- Run debugpy_unreal.start() to begin a debug session, using the same port that you will use to attach your debugger (5678 is the default for VS Code).
- Attach your debugger from VS Code (see the "launch.json" configuration below).
-
Run debugpy_unreal.breakpoint() to instruct the attached debugger to breakpoint on the next statement executed.
-
Import
debugpy_unreal
into Python within the UE Editor. - If debugpy has not yet been installed, run
debugpy_unreal.install_debugpy()
. - Run
debugpy_unreal.start()
to begin a debug session, using the same port that you will use to attach your debugger (5678 is the default for VS Code). - Attach your debugger from VS Code (see the "launch.json" configuration below).
- Run
debugpy_unreal.breakpoint()
to instruct the attached debugger to breakpoint on the next statement executed.
Steps¶
- Install the third-party library debugpy via pip. Use 3rd Python package
- Add a debug configuration file in VSCode: Main Menu > Run > Add Configuration > Python > Remote Connect, Hostname: localhost, Port: 5678, redirectOutput: true
{
"version": "0.2.0",
"configurations": [
{
"name": "Unreal Python",
"type": "python",
"request": "attach",
"connect": {
"host": "localhost",
"port": 5678
},
"redirectOutput": true
}
]
}
- When you need to debug, run the following commands in the UE Python command line console:
import debugpy_unreal
debugpy_unreal.start()
debugpy_unreal.start() calls debugpy.wait_for_client() to wait for VSCode, which may cause the editor to freeze. Don't worry, just proceed to the next step.
-
In VSCode, go to Main Menu > Run > Start Debugging (F5) to attach VSCode to UE's Python. Make sure you have enabled the debug configuration file created in step 2.
-
Open the .py file you want to debug in VSCode, set breakpoints, and run the code. You can also use
debugpy_unreal.breakpoint()
to directly interrupt Python execution in the .py file (the file will be opened automatically in VSCode).
Other Tips¶
In actual development, in addition to debugging in VSCode, you can use the following methods to quickly identify and fix errors:
Print method¶
Using print
or unreal.log
to display variable values can help you quickly identify errors.
NOTE
Python's logging module can be used for log output, but the display level in UE may be incorrect. For example, warn will appear as a red Error
Directly obtaining objects in the editor¶
Objects in Python, whether they are unreal
objects or objects within self
, such as self.data
, can be obtained and their properties can be viewed in the Unreal Engine Python command line console.
For example, in Get Object in Unreal Engine Editor, various UObject objects are assigned to the global variable _r, and their properties and methods can be viewed.
Additionally, if you want to directly modify or access the content in the interface, you can do so in the Python command line. In the following example, the Chameleon Tools object in the minimal example is obtained directly through the global variable name chameleon_example
, and the content of the TextBlock is modified.
chameleon_example.data.set_text("InfoOutput", "Some Text")
Reasonable assertions¶
Adding appropriate assertions in Python code can help developers quickly identify errors in the code.
For example, ensure an object is not None:
assert my_object, f"my_object is None."
For example, ensure the length or type of a variable meets expectations and requirements:
assert len(object_pair) == 2, f"object_pair len: {len(object_pair)} != 2"
And so on.